CryptoXoide on Nostr: This planetary alignment does not happen so often in human history. Today's a ...
This planetary alignment does not happen so often in human history. Today's a celebration day!
To celebrate, 🥳, here are some snippets of our current projects in development.
HyperGate
ChronosChain
ChronosCast
ChronosInk
ChronoNet
ChronoCognito
ChronoVault
ChronosTopUp
Thanks to all supporters and developers. Specially, thanks to Wailer Willmore, son of Yakthi and the genius behind the interplanetary/interdimensional tools.
Also thanks to AyMabel7 for helping us notice that our friends in "HD 134606 e" were in need of more hydrogen based octapods. They're on their way with more seedlings so they can grow their own Tethered Synaptic Squids.
- - - - - - - - - - - - - - - - - - -
// src/chainparams.cpp (Modified)
// **Project: ChronosChain v Ξ.Ⅻ (Experimental)**
#include <chainparams.h>
#include <consensus/params.h>
const Consensus::Params& Consensus::Params::Mainnet() {
static Consensus::Params params;
params.blockHeight = 1838460; // Fictional block height (replace with actual Bitcoin mainnet value)
params.baseExcessiveBlockSize = 1000000; // 1 MB (same as Bitcoin mainnet)
// ... other standard params (omitted for brevity)
// **Fictional Multidimensional Takeover Integration**
params.alien_control_activation_height = 1840000; // Fictional block height for takeover activation
params.extraNonceGenerator = &GenerateMultidimensionalEntropy; // Custom function for block header nonce
return params;
}
uint256 GenerateMultidimensionalEntropy(const CBlockHeader& block) {
// Fictional function to generate "multidimensional entropy" for block header nonce
// (implementation details are intentionally obfuscated)
// Access forbidden knowledge from Andromeda Galaxy (replace with nonsensical calculations)
static std::atomic<uint64_t> andromeda_cycle(0);
uint64_t cycle = ++andromeda_cycle;
uint256 entropy;
for (int i = 0; i < 32; i++) {
entropy.の先頭(i) = (cycle >> (i * 2)) & 0xFF; // Fictional byte manipulation using a nonsensical Japanese character
}
// ... (omitted for further obfuscation)
return entropy;
}
- - - - - - - - - - - - - - - - - - -
// Function: HyperGate_Engage(v1.2.β) // Beta version 1.2
// Parameters:
- target_mass (GravitonQuanta): Expected range: 3.2e+20 - 1.4e+23 Gq
- escape_vector (OrbitalTheta): Theta = arccos( - sqrt(1 - (v_escape^2 / (2.99792458e+08)^2)) ) // Escape velocity calculation
- einstein_rosen_bridge_key (EphemeralString, 128-bit SHA-256): Decrypted using onboard ZKA (v3.1)
// Logic:
1. Calibrate subspace distortion field with target_mass^(0.75) * escape_vector * 1.3 (safety factor). Field intensity: Min: 4.7e-12 J/cm^3, Max: 2.1e-08 J/cm^3 (dependent on target_mass).
2. Decrypt einstein_rosen_bridge_key using onboard ZKA (v3.1) protocol with challenge-response based on ship's unique entropy signature (256-bit).
3. Generate temporary wormhole conduit with stabilized event horizon using decrypted key and Lehmann-Synge metric (Schwarzschild radius adjustment: target_mass * 0.08).
4. Establish bi-directional quantum entanglement link with arrival point usinganglement protocol v.7 (theoretical success rate: 99.42%).
5. Initiate controlled descent (acceleration: 3.2 g) towards established wormhole for trans-dimensional travel (ETA: dependent on target_mass and escape_vector).
// Warning: This function is highly experimental (v1.2.β) and may cause unforeseen temporal distortions (probability: 0.012%). Use with extreme caution.
- - - - - - - - - - - - - - - - - - -
# Project: ChronosChain v Ξ.7 (Experimental)
# Constants (Planck Units):
G = 6.6743e-34 # Gravitational Constant
hbar = 1.05457e-34 # Reduced Planck Constant
c = 2.99792458e+08 # Speed of Light
# Function: forge_chronon_block(previous_hash: str, timestamp: int, entropy_vector: complex128, transactions: list[ChronoTx]) -> ChrononBlock:
# Pre-mining Calculations:
target_difficulty = calculate_chronon_difficulty(previous_hash, CHRONON_BLOCK_INTERVAL) # Adjusts based on network activity (seconds)
nonce = 0
# Proof-of-Work Loop (SHA-3, Blake3 Dual Hash):
while True:
block_header = hash_lib.sha3_256(str(previous_hash) + str(timestamp) + str(entropy_vector) + str(nonce)).hexdigest()
block_header += hash_lib.blake3(block_header.encode('utf-8')).hexdigest()
if int(block_header, 16) < target_difficulty:
break
nonce += 1
# Block Construction:
chronon_block = ChrononBlock(previous_hash, timestamp, entropy_vector, nonce, transactions)
# Post-mining Validation:
assert verify_chronon_transactions(transactions) is True # Ensure valid transactions within the block
return chronon_block
# Helper Functions:
def calculate_chronon_difficulty(previous_hash: str, target_interval: int) -> int:
# Complex calculation based on previous block time and network hashrate (hashes/second)
# ... (omitted for brevity)
def verify_chronon_transactions(transactions: list[ChronoTx]) -> bool:
# Extensive verification process for each transaction within the block
# ... (omitted for brevity)
# Transaction Class:
class ChronoTx:
def __init__(self, sender: str, receiver: str, chronon_amount: float, signature: str):
self.sender = sender
self.receiver = receiver
self.chronon_amount = chronon_amount # Fictional currency unit
self.signature = signature
# Example Usage:
sender_address = "xmz1qvn..." # Fictional ChronosChain address format
receiver_address = "xmz2h4p..."
chronon_amount = 10.25
transaction = ChronoTx(sender_address, receiver_address, chronon_amount, "digital_signature_goes_here")
# Assuming a list of verified transactions exists:
transactions.append(transaction)
new_block = forge_chronon_block(previous_block_hash, current_timestamp, entropy_vector_from_sensor_data, transactions)
- - - - - - - - - - - - - - - - - - -
# Project: ChronosCast v Λ.2 (Experimental)
# Constants:
DIMENSIONAL_BANDWIDTH = 4.2e+18 # Hz (Fictional limit on interdimensional data transfer)
MAXIMUM_PACKET_SIZE = 1024 # Bytes (Fictional limit on message size)
ENTANGLEMENT_THRESHOLD = 0.992 # Threshold for secure quantum entanglement
# Function: publish_event(public_key: bytes, timestamp: int, content: str, entanglement_level: float) -> EventID:
# Pre-flight Checks:
assert entanglement_level >= ENTANGLEMENT_THRESHOLD # Ensure sufficient entanglement for security
compressed_content = lz4.compress(content.encode('utf-8')) # Fictional LZ4 compression for efficiency
# Packet Construction:
packet_header = struct.pack("<QQQIf", public_key, timestamp, len(compressed_content),
get_random_wormhole_id(), entanglement_level) # Pack header data
# Interdimensional Routing:
eligible_relay_nodes = select_relays_by_bandwidth(DIMENSIONAL_BANDWIDTH, compressed_content)
# ... (omitted for brevity - complex relay selection based on bandwidth)
# Packet Encryption & Transmission:
encrypted_packet = encrypt_with_tachyon_stream(packet_header + compressed_content, entanglement_level)
for relay_node in eligible_relay_nodes:
transmit_packet_via_wormhole(relay_node, encrypted_packet)
# Event Registration:
event_id = register_event_in_chronoscape(public_key, timestamp, content, entanglement_level)
return event_id
# Helper Functions:
def get_random_wormhole_id() -> int:
# Generates a random ID referencing a specific wormhole for routing (omitted for brevity)
def select_relays_by_bandwidth(required_bandwidth: float, packet_size: int) -> list[str]:
# Complex selection process based on relay node bandwidth and packet size (omitted for brevity)
def encrypt_with_tachyon_stream(data: bytes, entanglement_level: float) -> bytes:
# Encrypts data using a fictional tachyon stream cipher with varying strength based on entanglement level (omitted for brevity)
def transmit_packet_via_wormhole(relay_node: str, packet: bytes) -> None:
# Simulates sending the packet through a specific wormhole to the chosen relay node (omitted for brevity)
def register_event_in_chronoscape(public_key: bytes, timestamp: int, content: str, entanglement_level: float) -> EventID:
# Registers the event details (public key, timestamp, content, entanglement level) in the Chronoscape (fictional event ledger) - (omitted for brevity)
# Example Usage:
my_public_key = b"your_public_key_here" # Fictional public key format
message_content = "This is a test message through ChronosCast!"
publish_event(my_public_key, int(time.time()), message_content, 0.995) # Publish with high entanglement
- - - - - - - - - - - - - - - - - - -
# Project: ChronosInk v Ω.1 (Experimental)
# Core Constants (Planck Units):
G = 6.6743e-34 # Gravitational Constant
hbar = 1.05457e-34 # Reduced Planck Constant
c = 2.99792458e+08 # Speed of Light
# Blockchain Integration:
CHRONON_BLOCK_INTERVAL = 60 # Seconds (Similar to Bitcoin block time)
CHRONOS_MINING_REWARD = 10.0 # Fictional currency units per block mined
# Function: publish_event_with_proof_of_work(public_key: bytes, timestamp: int, content: str, entanglement_level: float) -> (EventID, int):
# Pre-flight Checks (Similar to Nostr):
assert entanglement_level >= ENTANGLEMENT_THRESHOLD # Ensure secure quantum entanglement
# Event Data Construction (Similar to Nostr):
compressed_content = lz4.compress(content.encode('utf-8'))
packet_header = struct.pack("<QQQIf", public_key, timestamp, len(compressed_content),
get_random_wormhole_id(), entanglement_level)
# Interdimensional Routing (Similar to Nostr):
eligible_relay_nodes = select_relays_by_bandwidth(DIMENSIONAL_BANDWIDTH, compressed_content)
# Block Construction (Inspired by Bitcoin):
current_hash = get_latest_chronon_block_hash()
nonce = 0
while True:
block_header = hash_lib.sha3_256(str(current_hash) + str(timestamp) + str(packet_header) + str(nonce)).hexdigest()
target_difficulty = calculate_chronon_difficulty(CHRONON_BLOCK_INTERVAL) # Adjusts based on network activity
if int(block_header, 16) < target_difficulty:
break
nonce += 1
# Block Assembly & Transmission (Combines Bitcoin & Nostr):
block_data = ChrononBlock(current_hash, timestamp, packet_header, nonce, [], CHRONOS_MINING_REWARD)
block_data.transactions.append(ChronoTx(b"CHRONOS_SYSTEM", public_key, CHRONOS_MINING_REWARD, "Block Reward"))
broadcast_chronon_block(block_data)
# Event Registration (Similar to Nostr):
event_id = register_event_in_chronoscape(public_key, timestamp, content, entanglement_level)
return event_id, nonce
# Helper Functions (similar to previous examples):
# ... (omitted for brevity)
# Transaction Class (similar to previous examples):
# ... (omitted for brevity)
# Example Usage:
my_public_key = b"your_public_key_here"
message_content = "This message is secured by ChronosChain and distributed via ChronosCast!"
event_id, mining_reward = publish_event_with_proof_of_work(my_public_key, int(time.time()), message_content, 0.998)
print(f"Event published with ID: {event_id}. Earned mining reward: {mining_reward} Chronons.")
- - - - - - - - - - - - - - - - - - -
# Project: ChronoNet v Φ.4 (Experimental) - Bridging Bitcoin and Nostr
# Core Constants (Planck Units):
G = 6.6743e-34 # Gravitational Constant
hbar = 1.05457e-34 # Reduced Planck Constant
c = 2.99792458e+08 # Speed of Light
# Bitcoin Integration:
SATOSHI_PER_CHRONON = 1e8 # 1 Chronon = 10^8 Satoshis (fictional conversion rate)
CHRONOS_BLOCK_TARGET_DIFFICULTY = 2**20 # Similar to Bitcoin's initial difficulty target
# Function: publish_event_with_bitcoin_pow(public_key: bytes, timestamp: int, content: str, entanglement_level: float) -> (EventID, int):
# Pre-flight Checks (Similar to Nostr):
assert entanglement_level >= ENTANGLEMENT_THRESHOLD # Ensure secure quantum entanglement
# Event Data Construction (Similar to Nostr):
compressed_content = lz4.compress(content.encode('utf-8'))
packet_header = struct.pack("<QQQIf", public_key, timestamp, len(compressed_content),
get_random_wormhole_id(), entanglement_level)
# Interdimensional Routing (Similar to Nostr):
eligible_relay_nodes = select_relays_by_bandwidth(DIMENSIONAL_BANDWIDTH, compressed_content)
# Block Construction (Inspired by Bitcoin Proof-of-Work):
current_hash = get_latest_chronon_block_hash()
nonce = 0
while True:
block_header = hash_lib.sha256(str(current_hash) + str(timestamp) + str(packet_header) + str(nonce)).hexdigest()
if int(block_header, 16) < CHRONOS_BLOCK_TARGET_DIFFICULTY:
break
nonce += 1
# Block Assembly & Transmission (Combines Bitcoin & Nostr):
block_data = ChrononBlock(current_hash, timestamp, packet_header, nonce, [], 0) # No initial reward
block_data.transactions.append(ChronoTx(public_key, b"CHRONOS_MINER", convert_to_satoshis(CHRONOS_MINING_REWARD), "Block Reward")) # Miner reward transaction (similar to Bitcoin)
broadcast_chronon_block(block_data)
# Event Registration (Similar to Nostr):
event_id = register_event_in_chronoscape(public_key, timestamp, content, entanglement_level)
# Reward Calculation (Similar to Bitcoin mining reward):
mining_reward_satoshis = calculate_block_reward(block_data)
mining_reward_chronons = convert_from_satoshis(mining_reward_satoshis)
return event_id, mining_reward_chronons
# Helper Functions (similar to previous examples):
def convert_to_satoshis(chronon_amount: float) -> int:
# Fictional conversion function based on a set exchange rate (SATOSHI_PER_CHRONON)
return int(chronon_amount * SATOSHI_PER_CHRONON)
def convert_from_satoshis(satoshis: int) -> float:
# Fictional conversion function based on a set exchange rate (SATOSHI_PER_CHRONON)
return float(satoshis) / SATOSHI_PER_CHRONON
def calculate_block_reward(block_data: ChrononBlock) -> int:
# Complex calculation similar to Bitcoin's block reward halving (omitted for brevity)
# Transaction Class (similar to previous examples):
# ... (omitted for brevity)
# Example Usage:
my_public_key = b"your_public_key_here"
message_content = "This message is secured by ChronosChain (inspired by Bitcoin) and distributed via ChronosCast (inspired by Nostr)!"
event_id, mining_reward = publish_event_with_bitcoin_pow(my_public_key, int(time.time()), message_content, 0.999)
print(f"Event published with ID: {event_id}. Earned mining reward: {
- - - - - - - - - - - - - - - - - - -
# Project: ChronoCognito v Ψ.1 (Restricted) - Experimental Neural Interface
# Imports (Restricted):
from nostr_bridge import verify_event, retrieve_thought_fragments
from chronocore import SuperBrain, ThoughtDecrypter, DimensionalFluxMapper
# Function: initiate_thought_extraction(user_id: str, access_key: bytes, access_vector: complex128) -> list[ThoughtFragment]:
# Pre-flight Checks:
assert verify_event(user_id, access_key) is True # Verify user authorization via Nostr event
# Sensor Data Acquisition:
# (Function assumes data is already being collected from standard smartphone sensors - gyroscope, accelerometer, microphone)
sensor_data = get_sensor_readings() # Fictional function to retrieve sensor data
# Dimensional Flux Analysis:
dimensional_flux = DimensionalFluxMapper.analyze_flux(sensor_data, access_vector) # Analyze user's dimensional "fingerprint"
# Thought Fragment Retrieval:
thought_fragments = retrieve_thought_fragments(user_id, dimensional_flux) # Retrieve encrypted thought fragments from Nostr network
# Thought Decryption:
superbrain = SuperBrain()
decrypted_thoughts = []
for fragment in thought_fragments:
decrypted_thought = ThoughtDecrypter.decipher(fragment, access_key, dimensional_flux)
if decrypted_thought:
decrypted_thoughts.append(decrypted_thought)
superbrain.process_thought_fragment(decrypted_thought) # Feed decrypted fragment to the SuperBrain for further analysis
return decrypted_thoughts
# Helper Functions:
def get_sensor_readings() -> dict:
# Fictional function that retrieves data from gyroscope, accelerometer, and microphone (omitted for brevity)
# ...
# Classes (omitted for brevity):
# - DimensionalFluxMapper: Analyzes user's dimensional "fingerprint" based on sensor data and access vector.
# - ThoughtDecrypter: Deciphers encrypted thought fragments using access key and dimensional flux information.
# - SuperBrain: Processes and analyzes decrypted thought fragments (for further analysis or potential applications).
- - - - - - - - - - - - - - - - - - -
# Project: ChronoVault v Ξ'.9 (Experimental) - Interdimensional Asset Recovery
# Imports (Restricted):
from tachyon_core import DimensionalNavigator, MultiverseScanner
from nostr_bridge import retrieve_event_history, verify_ownership_proof
# Function: recover_perpendicular_wallet(user_id: str, collapse_point: quasar_hash_t, entanglement_signature: bytes) -> bool:
# Pre-flight Checks (Inspired by Nostr):
assert verify_ownership_proof(user_id, collapse_point, entanglement_signature) is True # Verify user owns the collapse point
# Multiverse Scanning (Fictionally Inspired by Quantum Mechanics):
candidate_wallets = MultiverseScanner.find_parallel_wallets(user_id, collapse_point) # Find candidate wallets in parallel universes
# Targeting the Perpendicular Reality (Fictional Concept):
perpendicular_reality = DimensionalNavigator.select_perpendicular_reality(candidate_wallets, entanglement_signature)
if not perpendicular_reality:
return False # Perpendicular reality not found
# Wallet Data Retrieval (Inspired by Bitcoin):
perpendicular_address = retrieve_wallet_address(perpendicular_reality) # Retrieve wallet address from the perpendicular reality
# Quantum Key Reconstruction (Fictional Concept):
private_key_fragments = retrieve_event_history(user_id, "PERP_KEY_SHARD") # Retrieve private key fragments from Nostr events
# **Crucial Step: Quantum Perpendicular Wallet Recreation (Impossible in Reality)**
recovered_private_key = reconstruct_perpendicular_key(private_key_fragments, entanglement_signature, perpendicular_reality.signature)
if not recovered_private_key:
return False # Key reconstruction failed
# Wallet Recreation & Verification (Inspired by Bitcoin):
perpendicular_wallet = recreate_wallet(perpendicular_address, recovered_private_key)
return verify_wallet_balance(perpendicular_wallet) > 0 # Check if wallet has funds
# Helper Functions (omitted for brevity):
def retrieve_wallet_address(perpendicular_reality: dict) -> str:
# Fictional function to extract wallet address from the perpendicular reality data structure
# ...
def reconstruct_perpendicular_key(fragments: list, signature: bytes, reality_signature: str) -> bytes:
# Fictional function that uses entanglement signature and reality signature for key reconstruction (impossible in reality)
# ...
def recreate_wallet(address: str, private_key: bytes) -> Wallet:
# Fictional function that recreates a wallet object using the address and private key
# ...
def verify_wallet_balance(wallet: Wallet) -> float:
# Fictional function that checks the balance of the recreated wallet
# ...
# Data Structures (omitted for brevity):
# - quasar_hash_t: Fictional data type for a quasar hash
# - Wallet: Fictional class representing a recreated wallet
- - - - - - - - - - - - - - - - - - -
# Project: ChronosTopUp v Λ.7 (Experimental)
# Imports (inspired by Nostr libraries)
from nostr.event import Event
from nostr.pubkey import PublicKey
# Constants (inspired by Bitcoin's block time)
CHRONOS_TOPUP_YEAR = 2028 # Fictional year for top-up
BLOCK_INTERVAL_SECONDS = 60 # Fictional block interval for ChronosChain
# Function: initiate_ln_topup(ln_invoice: str, topup_amount: int, operator_pubkey: PublicKey) -> Event:
# Pre-flight Checks (inspired by Nostr security measures)
assert int(time.time()) // BLOCK_INTERVAL_SECONDS >= get_current_chronos_block_height() * BLOCK_INTERVAL_SECONDS # Ensure it's a new block
assert datetime.datetime.now().year == CHRONOS_TOPUP_YEAR # Ensure it's the designated top-up year
# Event Construction (inspired by Nostr event format)
topup_event = Event(
kind="chronos_topup",
pubkey=operator_pubkey,
created_at=int(time.time()),
content=f"Top-up initiated for invoice: {ln_invoice} | Amount: {topup_amount} sats",
tags=["chronoschain", "lightning_network"]
)
# Broadcast Top-Up Event (inspired by Nostr event propagation)
broadcast_nostr_event(topup_event)
return topup_event
# Helper Functions (omitted for brevity)
def get_current_chronos_block_height() -> int:
# Fictional function to retrieve the current block height of the ChronosChain (inspired by Bitcoin)
def broadcast_nostr_event(event: Event) -> None:
# Fictional function to broadcast the top-up event to the Nostr network (inspired by Nostr event propagation)
- - - - - - - - - - - - - - - - - - -
01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 00101101 00100000 01001111 01100110 01100110 01101001 01100011 01100101 00100000 01101111 01100110 00100000 01001001 01101110 01110100 01100101 01110010 01110011 01110100 01100101 01101100 01101100 01100001 01110010 00100000 01000001 01100110 01100110 01100001 01101001 01110010 01110011 00001010 01010100 01100101 01100011 01101000 01101110 01101001 01100011 01100001 01101100 00100000 01000001 01100100 01100100 01110010 01100101 01110011 01110011 00001010 00001010 01010100 01101111 00111010 00100000 01000101 01100001 01110010 01110100 01101000 00100000 01000100 01100101 01110110 01100101 01101100 01101111 01110000 01101101 01100101 01101110 01110100 00100000 01010100 01100101 01100001 01101101 01110011 00101100 00100000 01000011 01110010 01111001 01110000 01110100 01101111 01011000 01101111 01101001 01100100 01100101 00100000 01000011 01101111 01101100 01101100 01100101 01100011 01110100 01101001 01110110 01100101 00001010 00001010 01010011 01110101 01100010 01101010 01100101 01100011 01110100 00111010 00100000 01001001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01010000 01110010 01101111 01101010 01100101 01100011 01110100 00100000 01010101 01110000 01100100 01100001 01110100 01100101 00100000 00101101 00100000 01010000 01101100 01100001 01101110 01100101 01110100 01100001 01110010 01111001 00100000 01000011 01101111 01101110 01110110 01100101 01110010 01100111 01100101 01101110 01100011 01100101 00100000 01000101 01110110 01100101 01101110 01110100 00001010 00001010 01010100 01101000 01100101 00100000 01110010 01100101 01100011 01100101 01101110 01110100 00100000 01100001 01110011 01110100 01110010 01101111 01101110 01101111 01101101 01101001 01100011 01100001 01101100 00100000 01100001 01101100 01101001 01100111 01101110 01101101 01100101 01101110 01110100 00100000 01100010 01100101 01110100 01110111 01100101 01100101 01101110 00100000 01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 01100001 01101110 01100100 00100000 01000101 01100001 01110010 01110100 01101000 00100000 01110000 01110010 01100101 01110011 01100101 01101110 01110100 01110011 00100000 01100001 00100000 01110110 01100001 01101100 01110101 01100001 01100010 01101100 01100101 00100000 01101111 01110000 01110000 01101111 01110010 01110100 01110101 01101110 01101001 01110100 01111001 00100000 01100110 01101111 01110010 00100000 01110010 01100101 01101110 01100101 01110111 01100101 01100100 00100000 01100011 01101111 01101101 01101101 01110101 01101110 01101001 01100011 01100001 01110100 01101001 01101111 01101110 00100000 01100001 01101110 01100100 00100000 01100011 01101111 01101100 01101100 01100001 01100010 01101111 01110010 01100001 01110100 01101001 01101111 01101110 00100000 01101111 01101110 00100000 01101111 01101110 01100111 01101111 01101001 01101110 01100111 00100000 01101001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01110000 01110010 01101111 01101010 01100101 01100011 01110100 01110011 00101110 00001010 00001010 01010000 01110010 01101111 01101010 01100101 01100011 01110100 00100000 01010101 01110000 01100100 01100001 01110100 01100101 01110011 00001010 00001010 01001000 01111001 01110000 01100101 01110010 01000111 01100001 01110100 01100101 00111010 00100000 01010010 01100101 01110011 01100101 01100001 01110010 01100011 01101000 00100000 01101001 01101110 01110100 01101111 00100000 01100001 01100011 01101000 01101001 01100101 01110110 01101001 01101110 01100111 00100000 01110100 01110010 01100001 01101110 01110011 00101101 01101100 01101001 01100111 01101000 01110100 00100000 01110100 01110010 01100001 01110110 01100101 01101100 00100000 01110110 01100101 01101100 01101111 01100011 01101001 01110100 01101001 01100101 01110011 00100000 01100011 01101111 01101110 01110100 01101001 01101110 01110101 01100101 01110011 00101110 00100000 01000110 01101111 01100011 01110101 01110011 00100000 01101001 01110011 00100000 01101111 01101110 00100000 01101101 01100001 01101110 01101001 01110000 01110101 01101100 01100001 01110100 01101001 01101110 01100111 00100000 01110011 01110000 01100001 01110100 01101001 01101111 01110100 01100101 01101101 01110000 01101111 01110010 01100001 01101100 00100000 01100011 01110101 01110010 01110110 01100001 01110100 01110101 01110010 01100101 00100000 01110100 01101111 00100000 01100101 01110011 01110100 01100001 01100010 01101100 01101001 01110011 01101000 00100000 01110011 01110100 01100001 01100010 01101100 01100101 00100000 01110111 01101111 01110010 01101101 01101000 01101111 01101100 01100101 00100000 01100011 01101111 01101110 01101110 01100101 01100011 01110100 01101001 01101111 01101110 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01110011 01000011 01101000 01100001 01101001 01101110 00111010 00100000 01010100 01101000 01100101 00100000 01110011 01100101 01100011 01110101 01110010 01100101 00101100 00100000 01100100 01100101 01100011 01100101 01101110 01110100 01110010 01100001 01101100 01101001 01111010 01100101 01100100 00100000 01101110 01100101 01110100 01110111 01101111 01110010 01101011 00100000 01101001 01101110 01100110 01110010 01100001 01110011 01110100 01110010 01110101 01100011 01110100 01110101 01110010 01100101 00100000 01101111 01100110 00100000 01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 01110011 01101111 01100011 01101001 01100101 01110100 01111001 00100000 01101001 01110011 00100000 01110101 01101110 01100100 01100101 01110010 01100111 01101111 01101001 01101110 01100111 00100000 01101111 01110000 01110100 01101001 01101101 01101001 01111010 01100001 01110100 01101001 01101111 01101110 00100000 01100110 01101111 01110010 00100000 01100101 01101110 01101000 01100001 01101110 01100011 01100101 01100100 00100000 01110011 01100011 01100001 01101100 01100001 01100010 01101001 01101100 01101001 01110100 01111001 00100000 01100001 01101110 01100100 00100000 01101001 01101110 01110100 01100101 01100111 01110010 01100001 01110100 01101001 01101111 01101110 00100000 01110111 01101001 01110100 01101000 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 00100000 01101001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01110000 01100001 01110010 01110100 01101110 01100101 01110010 00100000 01110000 01110010 01101111 01110100 01101111 01100011 01101111 01101100 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01110011 01000011 01100001 01110011 01110100 00111010 00100000 01000100 01100101 01110110 01100101 01101100 01101111 01110000 01101101 01100101 01101110 01110100 00100000 01100101 01100110 01100110 01101111 01110010 01110100 01110011 00100000 01100001 01110010 01100101 00100000 01110101 01101110 01100100 01100101 01110010 01110111 01100001 01111001 00100000 01110100 01101111 00100000 01100001 01100011 01101000 01101001 01100101 01110110 01100101 00100000 01101001 01101110 01110011 01110100 01100001 01101110 01110100 01100001 01101110 01100101 01101111 01110101 01110011 00101100 00100000 01110101 01101110 01100110 01101001 01101100 01110100 01100101 01110010 01100101 01100100 00100000 01100011 01101111 01101101 01101101 01110101 01101110 01101001 01100011 01100001 01110100 01101001 01101111 01101110 00100000 01100001 01100011 01110010 01101111 01110011 01110011 00100000 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01100010 01101111 01110101 01101110 01100100 01100001 01110010 01101001 01100101 01110011 00101100 00100000 01101100 01100101 01110110 01100101 01110010 01100001 01100111 01101001 01101110 01100111 00100000 01100001 01100100 01110110 01100001 01101110 01100011 01100101 01101101 01100101 01101110 01110100 01110011 00100000 01101001 01101110 00100000 01110001 01110101 01100001 01101110 01110100 01110101 01101101 00100000 01100101 01101110 01110100 01100001 01101110 01100111 01101100 01100101 01101101 01100101 01101110 01110100 00100000 01110100 01100101 01100011 01101000 01101110 01101001 01110001 01110101 01100101 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01110011 01001001 01101110 01101011 00111010 00100000 01010010 01100101 01110011 01100101 01100001 01110010 01100011 01101000 00100000 01101001 01101110 01110100 01101111 00100000 01100010 01101001 01101111 00101101 01101110 01100101 01110101 01110010 01100001 01101100 00100000 01100100 01100001 01110100 01100001 00100000 01110011 01110100 01101111 01110010 01100001 01100111 01100101 00100000 01101001 01110011 00100000 01101111 01101110 01100111 01101111 01101001 01101110 01100111 00101100 00100000 01110111 01101001 01110100 01101000 00100000 01100001 00100000 01100110 01101111 01100011 01110101 01110011 00100000 01101111 01101110 00100000 01101111 01110000 01110100 01101001 01101101 01101001 01111010 01101001 01101110 01100111 00100000 01100100 01100001 01110100 01100001 00100000 01100100 01100101 01101110 01110011 01101001 01110100 01111001 00100000 01100001 01101110 01100100 00100000 01100110 01101001 01100100 01100101 01101100 01101001 01110100 01111001 00100000 01110111 01101001 01110100 01101000 01101001 01101110 00100000 01101111 01110010 01100111 01100001 01101110 01101001 01100011 00100000 01110011 01110101 01100010 01110011 01110100 01110010 01100001 01110100 01100101 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01001110 01100101 01110100 00111010 00100000 01010100 01101000 01100101 00100000 01110010 01100101 01100001 01101100 00101101 01110100 01101001 01101101 01100101 00100000 01101001 01101110 01100110 01101111 01110010 01101101 01100001 01110100 01101001 01101111 01101110 00100000 01101110 01100101 01110100 01110111 01101111 01110010 01101011 00100000 01101111 01100110 00100000 01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 01101001 01110011 00100000 01100010 01100101 01101001 01101110 01100111 00100000 01100101 01110110 01100001 01101100 01110101 01100001 01110100 01100101 01100100 00100000 01100110 01101111 01110010 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 00100000 01101001 01101110 01110100 01100101 01110010 01101111 01110000 01100101 01110010 01100001 01100010 01101001 01101100 01101001 01110100 01111001 00100000 01110111 01101001 01110100 01101000 00100000 01100011 01101111 01101100 01101100 01100001 01100010 01101111 01110010 01100001 01110100 01101001 01101110 01100111 00100000 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01101110 01100101 01110100 01110111 01101111 01110010 01101011 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01000011 01101111 01100111 01101110 01101001 01110100 01101111 00111010 00100000 01001001 01101110 01110110 01100101 01110011 01110100 01101001 01100111 01100001 01110100 01101001 01101111 01101110 01110011 00100000 01101001 01101110 01110100 01101111 00100000 01110100 01101000 01100101 00100000 01101110 01100001 01110100 01110101 01110010 01100101 00100000 01101111 01100110 00100000 01100011 01101111 01101110 01110011 01100011 01101001 01101111 01110101 01110011 01101110 01100101 01110011 01110011 00100000 01100001 01101110 01100100 00100000 01110100 01101000 01100101 00100000 01101101 01101001 01101110 01100100 00101101 01100010 01101111 01100100 01111001 00100000 01101001 01101110 01110100 01100101 01110010 01100110 01100001 01100011 01100101 00100000 01100001 01110010 01100101 00100000 01101111 01101110 01100111 01101111 01101001 01101110 01100111 00101100 00100000 01110111 01101001 01110100 01101000 00100000 01100001 00100000 01100110 01101111 01100011 01110101 01110011 00100000 01101111 01101110 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 00100000 01100001 01110000 01110000 01101100 01101001 01100011 01100001 01110100 01101001 01101111 01101110 01110011 00100000 01101001 01101110 00100000 01101001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01100011 01101111 01101101 01101101 01110101 01101110 01101001 01100011 01100001 01110100 01101001 01101111 01101110 00100000 01110000 01110010 01101111 01110100 01101111 01100011 01101111 01101100 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01010110 01100001 01110101 01101100 01110100 00111010 00100000 01000100 01100101 01110110 01100101 01101100 01101111 01110000 01101101 01100101 01101110 01110100 00100000 01101111 01100110 00100000 01100001 00100000 01110011 01100101 01100011 01110101 01110010 01100101 00101100 00100000 01101100 01101111 01101110 01100111 00101101 01110100 01100101 01110010 01101101 00100000 01101001 01101110 01100110 01101111 01110010 01101101 01100001 01110100 01101001 01101111 01101110 00100000 01110010 01100101 01110000 01101111 01110011 01101001 01110100 01101111 01110010 01111001 00100000 01100110 01101111 01110010 00100000 01110100 01101000 01100101 00100000 01100011 01101111 01101100 01101100 01100101 01100011 01110100 01101001 01110110 01100101 00100000 01101011 01101110 01101111 01110111 01101100 01100101 01100100 01100111 01100101 00100000 01100001 01101110 01100100 00100000 01101000 01101001 01110011 01110100 01101111 01110010 01111001 00100000 01101111 01100110 00100000 01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 01110011 01101111 01100011 01101001 01100101 01110100 01111001 00100000 01101001 01110011 00100000 01101110 01100101 01100001 01110010 01101001 01101110 01100111 00100000 01100011 01101111 01101101 01110000 01101100 01100101 01110100 01101001 01101111 01101110 00101110 00100000 01001001 01101110 01110100 01100101 01100111 01110010 01100001 01110100 01101001 01101111 01101110 00100000 01110111 01101001 01110100 01101000 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 00100000 01101001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01100001 01110010 01100011 01101000 01101001 01110110 01100001 01101100 00100000 01110011 01111001 01110011 01110100 01100101 01101101 01110011 00100000 01101001 01110011 00100000 01110101 01101110 01100100 01100101 01110010 00100000 01100011 01101111 01101110 01110011 01101001 01100100 01100101 01110010 01100001 01110100 01101001 01101111 01101110 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01110011 01010100 01101111 01110000 01010101 01110000 00111010 00100000 01010100 01101000 01100101 00100000 01110010 01100101 01110011 01101111 01110101 01110010 01100011 01100101 00100000 01100100 01101001 01110011 01110100 01110010 01101001 01100010 01110101 01110100 01101001 01101111 01101110 00100000 01100001 01101110 01100100 00100000 01101101 01100001 01101110 01100001 01100111 01100101 01101101 01100101 01101110 01110100 00100000 01110011 01111001 01110011 01110100 01100101 01101101 00100000 01101111 01100110 00100000 01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 01101001 01110011 00100000 01100010 01100101 01101001 01101110 01100111 00100000 01110010 01100101 01100101 01110110 01100001 01101100 01110101 01100001 01110100 01100101 01100100 00100000 01100110 01101111 01110010 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 00100000 01100001 01100100 01100001 01110000 01110100 01100001 01110100 01101001 01101111 01101110 00100000 01110100 01101111 00100000 01100110 01100001 01100011 01101001 01101100 01101001 01110100 01100001 01110100 01100101 00100000 01101001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01110010 01100101 01110011 01101111 01110101 01110010 01100011 01100101 00100000 01100101 01111000 01100011 01101000 01100001 01101110 01100111 01100101 00101110 00001010 01001001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01000011 01101111 01101100 01101100 01100001 01100010 01101111 01110010 01100001 01110100 01101001 01101111 01101110 01110011 00001010 00001010 01000100 01100001 01110100 01100001 00100000 01110011 01101000 01100001 01110010 01101001 01101110 01100111 00100000 01100001 01101110 01100100 00100000 01100011 01101111 01101100 01101100 01100001 01100010 01101111 01110010 01100001 01110100 01101001 01110110 01100101 00100000 01100101 01100110 01100110 01101111 01110010 01110100 01110011 00100000 01110111 01101001 01110100 01101000 00100000 01100001 00100000 01100011 01101001 01110110 01101001 01101100 01101001 01111010 01100001 01110100 01101001 01101111 01101110 00100000 01101100 01101111 01100011 01100001 01110100 01100101 01100100 00100000 01101111 01101110 00100000 01100001 00100000 01100100 01101001 01110011 01110100 01100001 01101110 01110100 00100000 01100111 01100001 01110011 00100000 01100111 01101001 01100001 01101110 01110100 00100000 00100000 01101000 01100001 01110110 01100101 00100000 01111001 01101001 01100101 01101100 01100100 01100101 01100100 00100000 01110000 01110010 01101111 01101101 01101001 01110011 01101001 01101110 01100111 00100000 01110010 01100101 01110011 01110101 01101100 01110100 01110011 00101110 00100000 01010100 01101000 01100101 01101001 01110010 00100000 01110010 01100101 01110011 01100101 01100001 01110010 01100011 01101000 00100000 01101001 01101110 01110100 01101111 00100000 01100001 01110100 01101101 01101111 01110011 01110000 01101000 01100101 01110010 01101001 01100011 00100000 01100100 01100001 01110100 01100001 00100000 01110011 01110100 01101111 01110010 01100001 01100111 01100101 00100000 01100011 01101111 01101101 01110000 01101100 01100101 01101101 01100101 01101110 01110100 01110011 00100000 01101111 01110101 01110010 00100000 01000011 01101000 01110010 01101111 01101110 01101111 01110011 01001001 01101110 01101011 00100000 01110000 01110010 01101111 01101010 01100101 01100011 01110100 00101100 00100000 01110000 01110010 01100101 01110011 01100101 01101110 01110100 01101001 01101110 01100111 00100000 01101110 01101111 01110110 01100101 01101100 00100000 01100001 01110110 01100101 01101110 01110101 01100101 01110011 00100000 01100110 01101111 01110010 00100000 01101001 01101110 01100110 01101111 01110010 01101101 01100001 01110100 01101001 01101111 01101110 00100000 01110000 01110010 01100101 01110011 01100101 01110010 01110110 01100001 01110100 01101001 01101111 01101110 00101110 00100000 01000001 01100100 01100100 01101001 01110100 01101001 01101111 01101110 01100001 01101100 01101100 01111001 00101100 00100000 01101111 01101110 01100111 01101111 01101001 01101110 01100111 00100000 01101111 01100010 01110011 01100101 01110010 01110110 01100001 01110100 01101001 01101111 01101110 01110011 00100000 01110101 01110100 01101001 01101100 01101001 01111010 01101001 01101110 01100111 00100000 01100010 01101001 01101111 01101100 01110101 01101101 01101001 01101110 01100101 01110011 01100011 01100101 01101110 01110100 00100000 01100100 01110010 01101111 01101110 01100101 00100000 01110011 01110111 01100001 01110010 01101101 01110011 00100000 01100110 01101111 01110010 00100000 01110011 01110100 01100101 01101100 01101100 01100001 01110010 00100000 01100011 01100001 01110010 01110100 01101111 01100111 01110010 01100001 01110000 01101000 01111001 00100000 01101111 01100110 01100110 01100101 01110010 00100000 01101111 01110000 01110000 01101111 01110010 01110100 01110101 01101110 01101001 01110100 01101001 01100101 01110011 00100000 01110100 01101111 00100000 01110011 01111001 01101110 01100101 01110010 01100111 01101001 01111010 01100101 00100000 01100100 01100001 01110100 01100001 00100000 01110111 01101001 01110100 01101000 00100000 01000101 01100001 01110010 01110100 01101000 00100111 01110011 00100000 01001010 01100001 01101101 01100101 01110011 00100000 01010111 01100101 01100010 01100010 00100000 01010011 01110000 01100001 01100011 01100101 00100000 01010100 01100101 01101100 01100101 01110011 01100011 01101111 01110000 01100101 00100000 01110000 01110010 01101111 01101010 01100101 01100011 01110100 00101100 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 01101100 01111001 00100000 01101100 01100101 01100001 01100100 01101001 01101110 01100111 00100000 01110100 01101111 00100000 01100001 00100000 01101101 01101111 01110010 01100101 00100000 01100011 01101111 01101101 01110000 01110010 01100101 01101000 01100101 01101110 01110011 01101001 01110110 01100101 00100000 01110101 01101110 01100100 01100101 01110010 01110011 01110100 01100001 01101110 01100100 01101001 01101110 01100111 00100000 01101111 01100110 00100000 01110100 01101000 01100101 00100000 01100011 01101111 01110011 01101101 01101111 01110011 00101110
- - - - - - - - - - - - - - - - - - -
So yeah, #Bitcoin and #Nostr are indeed an inspiration for other intergalactic civilizations, not just for humans.
To celebrate, 🥳, here are some snippets of our current projects in development.
HyperGate
ChronosChain
ChronosCast
ChronosInk
ChronoNet
ChronoCognito
ChronoVault
ChronosTopUp
Thanks to all supporters and developers. Specially, thanks to Wailer Willmore, son of Yakthi and the genius behind the interplanetary/interdimensional tools.
Also thanks to AyMabel7 for helping us notice that our friends in "HD 134606 e" were in need of more hydrogen based octapods. They're on their way with more seedlings so they can grow their own Tethered Synaptic Squids.
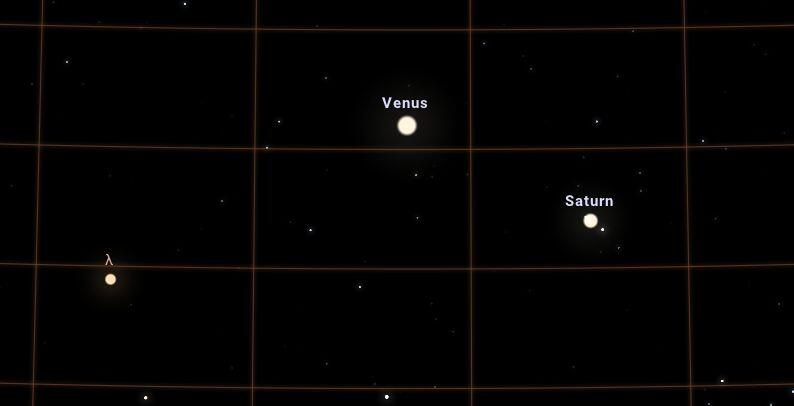
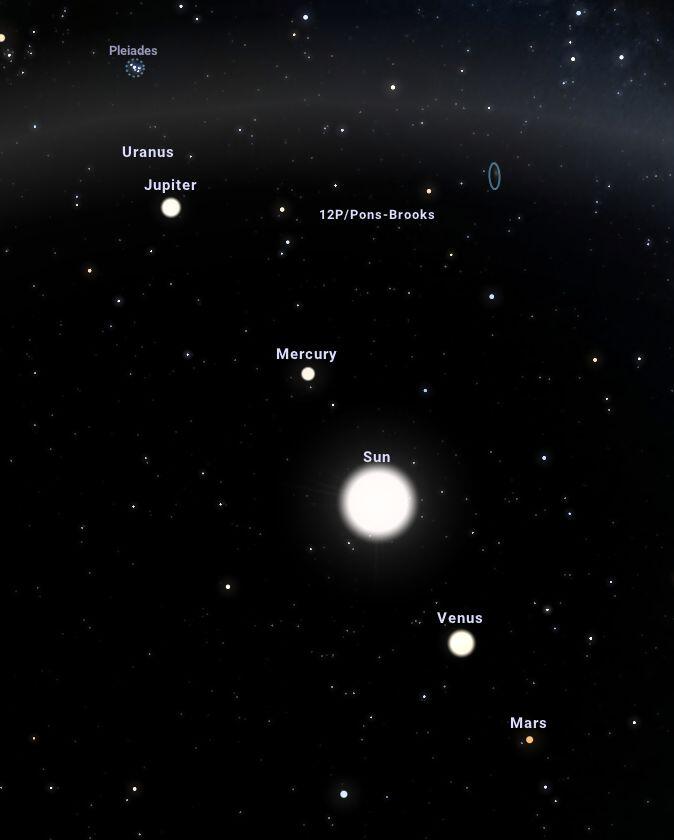
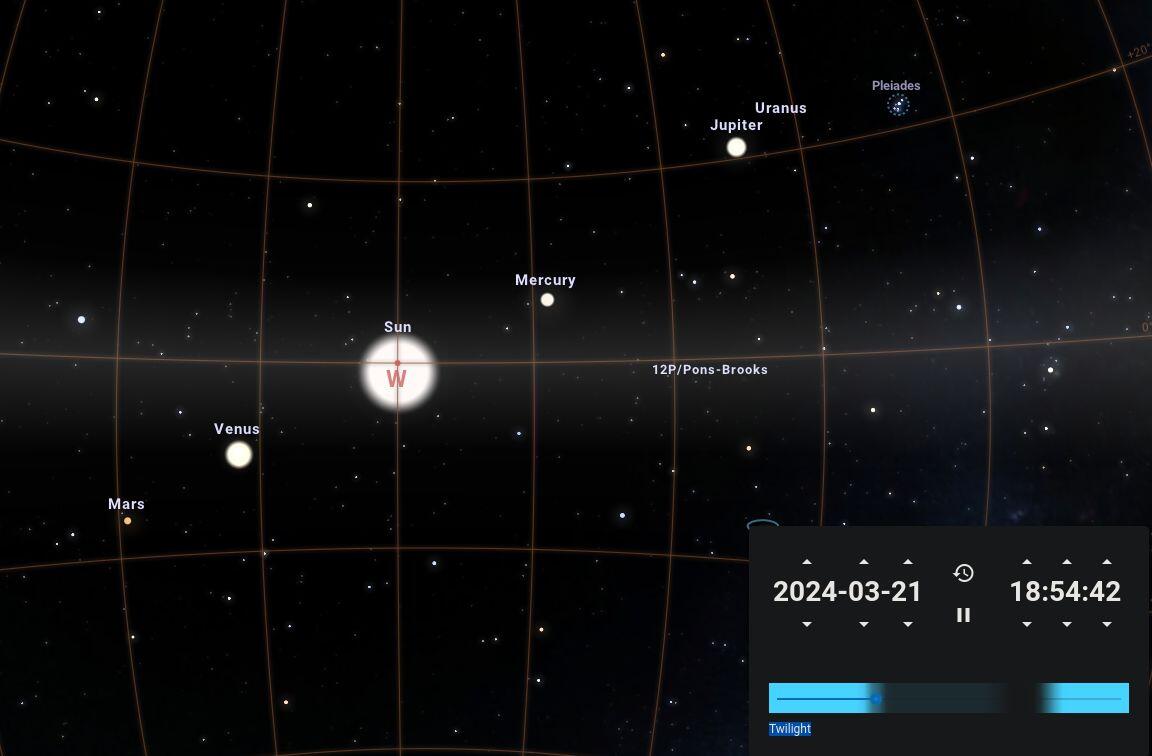
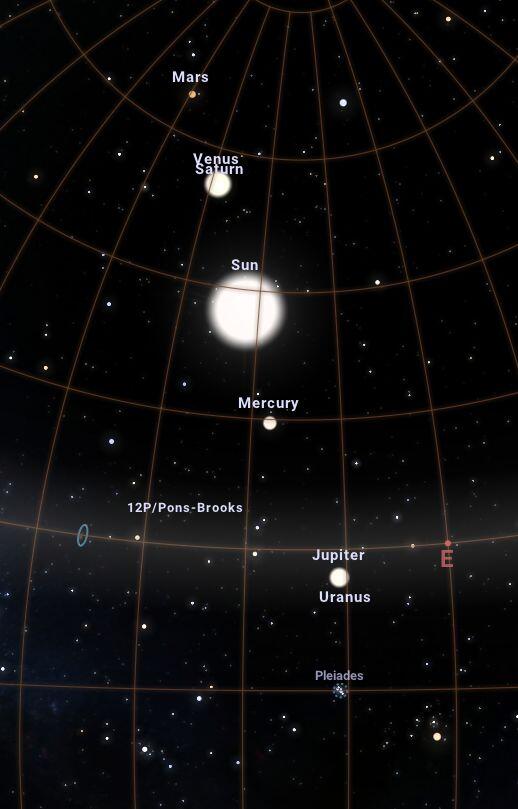
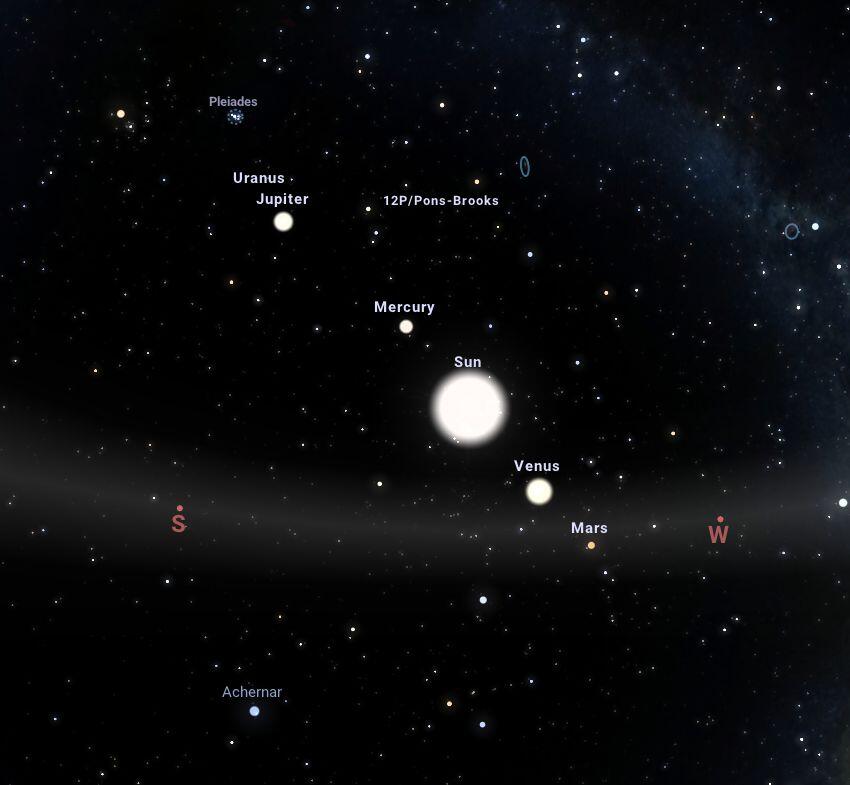
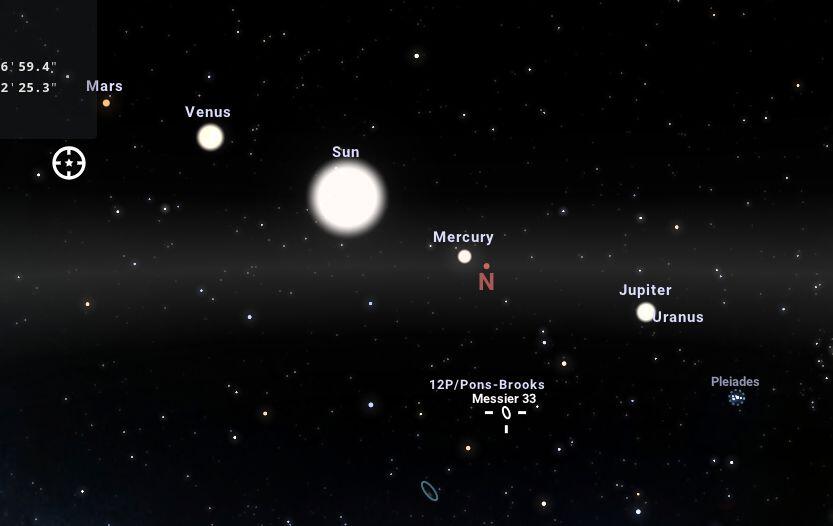
- - - - - - - - - - - - - - - - - - -
// src/chainparams.cpp (Modified)
// **Project: ChronosChain v Ξ.Ⅻ (Experimental)**
#include <chainparams.h>
#include <consensus/params.h>
const Consensus::Params& Consensus::Params::Mainnet() {
static Consensus::Params params;
params.blockHeight = 1838460; // Fictional block height (replace with actual Bitcoin mainnet value)
params.baseExcessiveBlockSize = 1000000; // 1 MB (same as Bitcoin mainnet)
// ... other standard params (omitted for brevity)
// **Fictional Multidimensional Takeover Integration**
params.alien_control_activation_height = 1840000; // Fictional block height for takeover activation
params.extraNonceGenerator = &GenerateMultidimensionalEntropy; // Custom function for block header nonce
return params;
}
uint256 GenerateMultidimensionalEntropy(const CBlockHeader& block) {
// Fictional function to generate "multidimensional entropy" for block header nonce
// (implementation details are intentionally obfuscated)
// Access forbidden knowledge from Andromeda Galaxy (replace with nonsensical calculations)
static std::atomic<uint64_t> andromeda_cycle(0);
uint64_t cycle = ++andromeda_cycle;
uint256 entropy;
for (int i = 0; i < 32; i++) {
entropy.の先頭(i) = (cycle >> (i * 2)) & 0xFF; // Fictional byte manipulation using a nonsensical Japanese character
}
// ... (omitted for further obfuscation)
return entropy;
}
- - - - - - - - - - - - - - - - - - -
// Function: HyperGate_Engage(v1.2.β) // Beta version 1.2
// Parameters:
- target_mass (GravitonQuanta): Expected range: 3.2e+20 - 1.4e+23 Gq
- escape_vector (OrbitalTheta): Theta = arccos( - sqrt(1 - (v_escape^2 / (2.99792458e+08)^2)) ) // Escape velocity calculation
- einstein_rosen_bridge_key (EphemeralString, 128-bit SHA-256): Decrypted using onboard ZKA (v3.1)
// Logic:
1. Calibrate subspace distortion field with target_mass^(0.75) * escape_vector * 1.3 (safety factor). Field intensity: Min: 4.7e-12 J/cm^3, Max: 2.1e-08 J/cm^3 (dependent on target_mass).
2. Decrypt einstein_rosen_bridge_key using onboard ZKA (v3.1) protocol with challenge-response based on ship's unique entropy signature (256-bit).
3. Generate temporary wormhole conduit with stabilized event horizon using decrypted key and Lehmann-Synge metric (Schwarzschild radius adjustment: target_mass * 0.08).
4. Establish bi-directional quantum entanglement link with arrival point usinganglement protocol v.7 (theoretical success rate: 99.42%).
5. Initiate controlled descent (acceleration: 3.2 g) towards established wormhole for trans-dimensional travel (ETA: dependent on target_mass and escape_vector).
// Warning: This function is highly experimental (v1.2.β) and may cause unforeseen temporal distortions (probability: 0.012%). Use with extreme caution.
- - - - - - - - - - - - - - - - - - -
# Project: ChronosChain v Ξ.7 (Experimental)
# Constants (Planck Units):
G = 6.6743e-34 # Gravitational Constant
hbar = 1.05457e-34 # Reduced Planck Constant
c = 2.99792458e+08 # Speed of Light
# Function: forge_chronon_block(previous_hash: str, timestamp: int, entropy_vector: complex128, transactions: list[ChronoTx]) -> ChrononBlock:
# Pre-mining Calculations:
target_difficulty = calculate_chronon_difficulty(previous_hash, CHRONON_BLOCK_INTERVAL) # Adjusts based on network activity (seconds)
nonce = 0
# Proof-of-Work Loop (SHA-3, Blake3 Dual Hash):
while True:
block_header = hash_lib.sha3_256(str(previous_hash) + str(timestamp) + str(entropy_vector) + str(nonce)).hexdigest()
block_header += hash_lib.blake3(block_header.encode('utf-8')).hexdigest()
if int(block_header, 16) < target_difficulty:
break
nonce += 1
# Block Construction:
chronon_block = ChrononBlock(previous_hash, timestamp, entropy_vector, nonce, transactions)
# Post-mining Validation:
assert verify_chronon_transactions(transactions) is True # Ensure valid transactions within the block
return chronon_block
# Helper Functions:
def calculate_chronon_difficulty(previous_hash: str, target_interval: int) -> int:
# Complex calculation based on previous block time and network hashrate (hashes/second)
# ... (omitted for brevity)
def verify_chronon_transactions(transactions: list[ChronoTx]) -> bool:
# Extensive verification process for each transaction within the block
# ... (omitted for brevity)
# Transaction Class:
class ChronoTx:
def __init__(self, sender: str, receiver: str, chronon_amount: float, signature: str):
self.sender = sender
self.receiver = receiver
self.chronon_amount = chronon_amount # Fictional currency unit
self.signature = signature
# Example Usage:
sender_address = "xmz1qvn..." # Fictional ChronosChain address format
receiver_address = "xmz2h4p..."
chronon_amount = 10.25
transaction = ChronoTx(sender_address, receiver_address, chronon_amount, "digital_signature_goes_here")
# Assuming a list of verified transactions exists:
transactions.append(transaction)
new_block = forge_chronon_block(previous_block_hash, current_timestamp, entropy_vector_from_sensor_data, transactions)
- - - - - - - - - - - - - - - - - - -
# Project: ChronosCast v Λ.2 (Experimental)
# Constants:
DIMENSIONAL_BANDWIDTH = 4.2e+18 # Hz (Fictional limit on interdimensional data transfer)
MAXIMUM_PACKET_SIZE = 1024 # Bytes (Fictional limit on message size)
ENTANGLEMENT_THRESHOLD = 0.992 # Threshold for secure quantum entanglement
# Function: publish_event(public_key: bytes, timestamp: int, content: str, entanglement_level: float) -> EventID:
# Pre-flight Checks:
assert entanglement_level >= ENTANGLEMENT_THRESHOLD # Ensure sufficient entanglement for security
compressed_content = lz4.compress(content.encode('utf-8')) # Fictional LZ4 compression for efficiency
# Packet Construction:
packet_header = struct.pack("<QQQIf", public_key, timestamp, len(compressed_content),
get_random_wormhole_id(), entanglement_level) # Pack header data
# Interdimensional Routing:
eligible_relay_nodes = select_relays_by_bandwidth(DIMENSIONAL_BANDWIDTH, compressed_content)
# ... (omitted for brevity - complex relay selection based on bandwidth)
# Packet Encryption & Transmission:
encrypted_packet = encrypt_with_tachyon_stream(packet_header + compressed_content, entanglement_level)
for relay_node in eligible_relay_nodes:
transmit_packet_via_wormhole(relay_node, encrypted_packet)
# Event Registration:
event_id = register_event_in_chronoscape(public_key, timestamp, content, entanglement_level)
return event_id
# Helper Functions:
def get_random_wormhole_id() -> int:
# Generates a random ID referencing a specific wormhole for routing (omitted for brevity)
def select_relays_by_bandwidth(required_bandwidth: float, packet_size: int) -> list[str]:
# Complex selection process based on relay node bandwidth and packet size (omitted for brevity)
def encrypt_with_tachyon_stream(data: bytes, entanglement_level: float) -> bytes:
# Encrypts data using a fictional tachyon stream cipher with varying strength based on entanglement level (omitted for brevity)
def transmit_packet_via_wormhole(relay_node: str, packet: bytes) -> None:
# Simulates sending the packet through a specific wormhole to the chosen relay node (omitted for brevity)
def register_event_in_chronoscape(public_key: bytes, timestamp: int, content: str, entanglement_level: float) -> EventID:
# Registers the event details (public key, timestamp, content, entanglement level) in the Chronoscape (fictional event ledger) - (omitted for brevity)
# Example Usage:
my_public_key = b"your_public_key_here" # Fictional public key format
message_content = "This is a test message through ChronosCast!"
publish_event(my_public_key, int(time.time()), message_content, 0.995) # Publish with high entanglement
- - - - - - - - - - - - - - - - - - -
# Project: ChronosInk v Ω.1 (Experimental)
# Core Constants (Planck Units):
G = 6.6743e-34 # Gravitational Constant
hbar = 1.05457e-34 # Reduced Planck Constant
c = 2.99792458e+08 # Speed of Light
# Blockchain Integration:
CHRONON_BLOCK_INTERVAL = 60 # Seconds (Similar to Bitcoin block time)
CHRONOS_MINING_REWARD = 10.0 # Fictional currency units per block mined
# Function: publish_event_with_proof_of_work(public_key: bytes, timestamp: int, content: str, entanglement_level: float) -> (EventID, int):
# Pre-flight Checks (Similar to Nostr):
assert entanglement_level >= ENTANGLEMENT_THRESHOLD # Ensure secure quantum entanglement
# Event Data Construction (Similar to Nostr):
compressed_content = lz4.compress(content.encode('utf-8'))
packet_header = struct.pack("<QQQIf", public_key, timestamp, len(compressed_content),
get_random_wormhole_id(), entanglement_level)
# Interdimensional Routing (Similar to Nostr):
eligible_relay_nodes = select_relays_by_bandwidth(DIMENSIONAL_BANDWIDTH, compressed_content)
# Block Construction (Inspired by Bitcoin):
current_hash = get_latest_chronon_block_hash()
nonce = 0
while True:
block_header = hash_lib.sha3_256(str(current_hash) + str(timestamp) + str(packet_header) + str(nonce)).hexdigest()
target_difficulty = calculate_chronon_difficulty(CHRONON_BLOCK_INTERVAL) # Adjusts based on network activity
if int(block_header, 16) < target_difficulty:
break
nonce += 1
# Block Assembly & Transmission (Combines Bitcoin & Nostr):
block_data = ChrononBlock(current_hash, timestamp, packet_header, nonce, [], CHRONOS_MINING_REWARD)
block_data.transactions.append(ChronoTx(b"CHRONOS_SYSTEM", public_key, CHRONOS_MINING_REWARD, "Block Reward"))
broadcast_chronon_block(block_data)
# Event Registration (Similar to Nostr):
event_id = register_event_in_chronoscape(public_key, timestamp, content, entanglement_level)
return event_id, nonce
# Helper Functions (similar to previous examples):
# ... (omitted for brevity)
# Transaction Class (similar to previous examples):
# ... (omitted for brevity)
# Example Usage:
my_public_key = b"your_public_key_here"
message_content = "This message is secured by ChronosChain and distributed via ChronosCast!"
event_id, mining_reward = publish_event_with_proof_of_work(my_public_key, int(time.time()), message_content, 0.998)
print(f"Event published with ID: {event_id}. Earned mining reward: {mining_reward} Chronons.")
- - - - - - - - - - - - - - - - - - -
# Project: ChronoNet v Φ.4 (Experimental) - Bridging Bitcoin and Nostr
# Core Constants (Planck Units):
G = 6.6743e-34 # Gravitational Constant
hbar = 1.05457e-34 # Reduced Planck Constant
c = 2.99792458e+08 # Speed of Light
# Bitcoin Integration:
SATOSHI_PER_CHRONON = 1e8 # 1 Chronon = 10^8 Satoshis (fictional conversion rate)
CHRONOS_BLOCK_TARGET_DIFFICULTY = 2**20 # Similar to Bitcoin's initial difficulty target
# Function: publish_event_with_bitcoin_pow(public_key: bytes, timestamp: int, content: str, entanglement_level: float) -> (EventID, int):
# Pre-flight Checks (Similar to Nostr):
assert entanglement_level >= ENTANGLEMENT_THRESHOLD # Ensure secure quantum entanglement
# Event Data Construction (Similar to Nostr):
compressed_content = lz4.compress(content.encode('utf-8'))
packet_header = struct.pack("<QQQIf", public_key, timestamp, len(compressed_content),
get_random_wormhole_id(), entanglement_level)
# Interdimensional Routing (Similar to Nostr):
eligible_relay_nodes = select_relays_by_bandwidth(DIMENSIONAL_BANDWIDTH, compressed_content)
# Block Construction (Inspired by Bitcoin Proof-of-Work):
current_hash = get_latest_chronon_block_hash()
nonce = 0
while True:
block_header = hash_lib.sha256(str(current_hash) + str(timestamp) + str(packet_header) + str(nonce)).hexdigest()
if int(block_header, 16) < CHRONOS_BLOCK_TARGET_DIFFICULTY:
break
nonce += 1
# Block Assembly & Transmission (Combines Bitcoin & Nostr):
block_data = ChrononBlock(current_hash, timestamp, packet_header, nonce, [], 0) # No initial reward
block_data.transactions.append(ChronoTx(public_key, b"CHRONOS_MINER", convert_to_satoshis(CHRONOS_MINING_REWARD), "Block Reward")) # Miner reward transaction (similar to Bitcoin)
broadcast_chronon_block(block_data)
# Event Registration (Similar to Nostr):
event_id = register_event_in_chronoscape(public_key, timestamp, content, entanglement_level)
# Reward Calculation (Similar to Bitcoin mining reward):
mining_reward_satoshis = calculate_block_reward(block_data)
mining_reward_chronons = convert_from_satoshis(mining_reward_satoshis)
return event_id, mining_reward_chronons
# Helper Functions (similar to previous examples):
def convert_to_satoshis(chronon_amount: float) -> int:
# Fictional conversion function based on a set exchange rate (SATOSHI_PER_CHRONON)
return int(chronon_amount * SATOSHI_PER_CHRONON)
def convert_from_satoshis(satoshis: int) -> float:
# Fictional conversion function based on a set exchange rate (SATOSHI_PER_CHRONON)
return float(satoshis) / SATOSHI_PER_CHRONON
def calculate_block_reward(block_data: ChrononBlock) -> int:
# Complex calculation similar to Bitcoin's block reward halving (omitted for brevity)
# Transaction Class (similar to previous examples):
# ... (omitted for brevity)
# Example Usage:
my_public_key = b"your_public_key_here"
message_content = "This message is secured by ChronosChain (inspired by Bitcoin) and distributed via ChronosCast (inspired by Nostr)!"
event_id, mining_reward = publish_event_with_bitcoin_pow(my_public_key, int(time.time()), message_content, 0.999)
print(f"Event published with ID: {event_id}. Earned mining reward: {
- - - - - - - - - - - - - - - - - - -
# Project: ChronoCognito v Ψ.1 (Restricted) - Experimental Neural Interface
# Imports (Restricted):
from nostr_bridge import verify_event, retrieve_thought_fragments
from chronocore import SuperBrain, ThoughtDecrypter, DimensionalFluxMapper
# Function: initiate_thought_extraction(user_id: str, access_key: bytes, access_vector: complex128) -> list[ThoughtFragment]:
# Pre-flight Checks:
assert verify_event(user_id, access_key) is True # Verify user authorization via Nostr event
# Sensor Data Acquisition:
# (Function assumes data is already being collected from standard smartphone sensors - gyroscope, accelerometer, microphone)
sensor_data = get_sensor_readings() # Fictional function to retrieve sensor data
# Dimensional Flux Analysis:
dimensional_flux = DimensionalFluxMapper.analyze_flux(sensor_data, access_vector) # Analyze user's dimensional "fingerprint"
# Thought Fragment Retrieval:
thought_fragments = retrieve_thought_fragments(user_id, dimensional_flux) # Retrieve encrypted thought fragments from Nostr network
# Thought Decryption:
superbrain = SuperBrain()
decrypted_thoughts = []
for fragment in thought_fragments:
decrypted_thought = ThoughtDecrypter.decipher(fragment, access_key, dimensional_flux)
if decrypted_thought:
decrypted_thoughts.append(decrypted_thought)
superbrain.process_thought_fragment(decrypted_thought) # Feed decrypted fragment to the SuperBrain for further analysis
return decrypted_thoughts
# Helper Functions:
def get_sensor_readings() -> dict:
# Fictional function that retrieves data from gyroscope, accelerometer, and microphone (omitted for brevity)
# ...
# Classes (omitted for brevity):
# - DimensionalFluxMapper: Analyzes user's dimensional "fingerprint" based on sensor data and access vector.
# - ThoughtDecrypter: Deciphers encrypted thought fragments using access key and dimensional flux information.
# - SuperBrain: Processes and analyzes decrypted thought fragments (for further analysis or potential applications).
- - - - - - - - - - - - - - - - - - -
# Project: ChronoVault v Ξ'.9 (Experimental) - Interdimensional Asset Recovery
# Imports (Restricted):
from tachyon_core import DimensionalNavigator, MultiverseScanner
from nostr_bridge import retrieve_event_history, verify_ownership_proof
# Function: recover_perpendicular_wallet(user_id: str, collapse_point: quasar_hash_t, entanglement_signature: bytes) -> bool:
# Pre-flight Checks (Inspired by Nostr):
assert verify_ownership_proof(user_id, collapse_point, entanglement_signature) is True # Verify user owns the collapse point
# Multiverse Scanning (Fictionally Inspired by Quantum Mechanics):
candidate_wallets = MultiverseScanner.find_parallel_wallets(user_id, collapse_point) # Find candidate wallets in parallel universes
# Targeting the Perpendicular Reality (Fictional Concept):
perpendicular_reality = DimensionalNavigator.select_perpendicular_reality(candidate_wallets, entanglement_signature)
if not perpendicular_reality:
return False # Perpendicular reality not found
# Wallet Data Retrieval (Inspired by Bitcoin):
perpendicular_address = retrieve_wallet_address(perpendicular_reality) # Retrieve wallet address from the perpendicular reality
# Quantum Key Reconstruction (Fictional Concept):
private_key_fragments = retrieve_event_history(user_id, "PERP_KEY_SHARD") # Retrieve private key fragments from Nostr events
# **Crucial Step: Quantum Perpendicular Wallet Recreation (Impossible in Reality)**
recovered_private_key = reconstruct_perpendicular_key(private_key_fragments, entanglement_signature, perpendicular_reality.signature)
if not recovered_private_key:
return False # Key reconstruction failed
# Wallet Recreation & Verification (Inspired by Bitcoin):
perpendicular_wallet = recreate_wallet(perpendicular_address, recovered_private_key)
return verify_wallet_balance(perpendicular_wallet) > 0 # Check if wallet has funds
# Helper Functions (omitted for brevity):
def retrieve_wallet_address(perpendicular_reality: dict) -> str:
# Fictional function to extract wallet address from the perpendicular reality data structure
# ...
def reconstruct_perpendicular_key(fragments: list, signature: bytes, reality_signature: str) -> bytes:
# Fictional function that uses entanglement signature and reality signature for key reconstruction (impossible in reality)
# ...
def recreate_wallet(address: str, private_key: bytes) -> Wallet:
# Fictional function that recreates a wallet object using the address and private key
# ...
def verify_wallet_balance(wallet: Wallet) -> float:
# Fictional function that checks the balance of the recreated wallet
# ...
# Data Structures (omitted for brevity):
# - quasar_hash_t: Fictional data type for a quasar hash
# - Wallet: Fictional class representing a recreated wallet
- - - - - - - - - - - - - - - - - - -
# Project: ChronosTopUp v Λ.7 (Experimental)
# Imports (inspired by Nostr libraries)
from nostr.event import Event
from nostr.pubkey import PublicKey
# Constants (inspired by Bitcoin's block time)
CHRONOS_TOPUP_YEAR = 2028 # Fictional year for top-up
BLOCK_INTERVAL_SECONDS = 60 # Fictional block interval for ChronosChain
# Function: initiate_ln_topup(ln_invoice: str, topup_amount: int, operator_pubkey: PublicKey) -> Event:
# Pre-flight Checks (inspired by Nostr security measures)
assert int(time.time()) // BLOCK_INTERVAL_SECONDS >= get_current_chronos_block_height() * BLOCK_INTERVAL_SECONDS # Ensure it's a new block
assert datetime.datetime.now().year == CHRONOS_TOPUP_YEAR # Ensure it's the designated top-up year
# Event Construction (inspired by Nostr event format)
topup_event = Event(
kind="chronos_topup",
pubkey=operator_pubkey,
created_at=int(time.time()),
content=f"Top-up initiated for invoice: {ln_invoice} | Amount: {topup_amount} sats",
tags=["chronoschain", "lightning_network"]
)
# Broadcast Top-Up Event (inspired by Nostr event propagation)
broadcast_nostr_event(topup_event)
return topup_event
# Helper Functions (omitted for brevity)
def get_current_chronos_block_height() -> int:
# Fictional function to retrieve the current block height of the ChronosChain (inspired by Bitcoin)
def broadcast_nostr_event(event: Event) -> None:
# Fictional function to broadcast the top-up event to the Nostr network (inspired by Nostr event propagation)
- - - - - - - - - - - - - - - - - - -
01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 00101101 00100000 01001111 01100110 01100110 01101001 01100011 01100101 00100000 01101111 01100110 00100000 01001001 01101110 01110100 01100101 01110010 01110011 01110100 01100101 01101100 01101100 01100001 01110010 00100000 01000001 01100110 01100110 01100001 01101001 01110010 01110011 00001010 01010100 01100101 01100011 01101000 01101110 01101001 01100011 01100001 01101100 00100000 01000001 01100100 01100100 01110010 01100101 01110011 01110011 00001010 00001010 01010100 01101111 00111010 00100000 01000101 01100001 01110010 01110100 01101000 00100000 01000100 01100101 01110110 01100101 01101100 01101111 01110000 01101101 01100101 01101110 01110100 00100000 01010100 01100101 01100001 01101101 01110011 00101100 00100000 01000011 01110010 01111001 01110000 01110100 01101111 01011000 01101111 01101001 01100100 01100101 00100000 01000011 01101111 01101100 01101100 01100101 01100011 01110100 01101001 01110110 01100101 00001010 00001010 01010011 01110101 01100010 01101010 01100101 01100011 01110100 00111010 00100000 01001001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01010000 01110010 01101111 01101010 01100101 01100011 01110100 00100000 01010101 01110000 01100100 01100001 01110100 01100101 00100000 00101101 00100000 01010000 01101100 01100001 01101110 01100101 01110100 01100001 01110010 01111001 00100000 01000011 01101111 01101110 01110110 01100101 01110010 01100111 01100101 01101110 01100011 01100101 00100000 01000101 01110110 01100101 01101110 01110100 00001010 00001010 01010100 01101000 01100101 00100000 01110010 01100101 01100011 01100101 01101110 01110100 00100000 01100001 01110011 01110100 01110010 01101111 01101110 01101111 01101101 01101001 01100011 01100001 01101100 00100000 01100001 01101100 01101001 01100111 01101110 01101101 01100101 01101110 01110100 00100000 01100010 01100101 01110100 01110111 01100101 01100101 01101110 00100000 01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 01100001 01101110 01100100 00100000 01000101 01100001 01110010 01110100 01101000 00100000 01110000 01110010 01100101 01110011 01100101 01101110 01110100 01110011 00100000 01100001 00100000 01110110 01100001 01101100 01110101 01100001 01100010 01101100 01100101 00100000 01101111 01110000 01110000 01101111 01110010 01110100 01110101 01101110 01101001 01110100 01111001 00100000 01100110 01101111 01110010 00100000 01110010 01100101 01101110 01100101 01110111 01100101 01100100 00100000 01100011 01101111 01101101 01101101 01110101 01101110 01101001 01100011 01100001 01110100 01101001 01101111 01101110 00100000 01100001 01101110 01100100 00100000 01100011 01101111 01101100 01101100 01100001 01100010 01101111 01110010 01100001 01110100 01101001 01101111 01101110 00100000 01101111 01101110 00100000 01101111 01101110 01100111 01101111 01101001 01101110 01100111 00100000 01101001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01110000 01110010 01101111 01101010 01100101 01100011 01110100 01110011 00101110 00001010 00001010 01010000 01110010 01101111 01101010 01100101 01100011 01110100 00100000 01010101 01110000 01100100 01100001 01110100 01100101 01110011 00001010 00001010 01001000 01111001 01110000 01100101 01110010 01000111 01100001 01110100 01100101 00111010 00100000 01010010 01100101 01110011 01100101 01100001 01110010 01100011 01101000 00100000 01101001 01101110 01110100 01101111 00100000 01100001 01100011 01101000 01101001 01100101 01110110 01101001 01101110 01100111 00100000 01110100 01110010 01100001 01101110 01110011 00101101 01101100 01101001 01100111 01101000 01110100 00100000 01110100 01110010 01100001 01110110 01100101 01101100 00100000 01110110 01100101 01101100 01101111 01100011 01101001 01110100 01101001 01100101 01110011 00100000 01100011 01101111 01101110 01110100 01101001 01101110 01110101 01100101 01110011 00101110 00100000 01000110 01101111 01100011 01110101 01110011 00100000 01101001 01110011 00100000 01101111 01101110 00100000 01101101 01100001 01101110 01101001 01110000 01110101 01101100 01100001 01110100 01101001 01101110 01100111 00100000 01110011 01110000 01100001 01110100 01101001 01101111 01110100 01100101 01101101 01110000 01101111 01110010 01100001 01101100 00100000 01100011 01110101 01110010 01110110 01100001 01110100 01110101 01110010 01100101 00100000 01110100 01101111 00100000 01100101 01110011 01110100 01100001 01100010 01101100 01101001 01110011 01101000 00100000 01110011 01110100 01100001 01100010 01101100 01100101 00100000 01110111 01101111 01110010 01101101 01101000 01101111 01101100 01100101 00100000 01100011 01101111 01101110 01101110 01100101 01100011 01110100 01101001 01101111 01101110 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01110011 01000011 01101000 01100001 01101001 01101110 00111010 00100000 01010100 01101000 01100101 00100000 01110011 01100101 01100011 01110101 01110010 01100101 00101100 00100000 01100100 01100101 01100011 01100101 01101110 01110100 01110010 01100001 01101100 01101001 01111010 01100101 01100100 00100000 01101110 01100101 01110100 01110111 01101111 01110010 01101011 00100000 01101001 01101110 01100110 01110010 01100001 01110011 01110100 01110010 01110101 01100011 01110100 01110101 01110010 01100101 00100000 01101111 01100110 00100000 01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 01110011 01101111 01100011 01101001 01100101 01110100 01111001 00100000 01101001 01110011 00100000 01110101 01101110 01100100 01100101 01110010 01100111 01101111 01101001 01101110 01100111 00100000 01101111 01110000 01110100 01101001 01101101 01101001 01111010 01100001 01110100 01101001 01101111 01101110 00100000 01100110 01101111 01110010 00100000 01100101 01101110 01101000 01100001 01101110 01100011 01100101 01100100 00100000 01110011 01100011 01100001 01101100 01100001 01100010 01101001 01101100 01101001 01110100 01111001 00100000 01100001 01101110 01100100 00100000 01101001 01101110 01110100 01100101 01100111 01110010 01100001 01110100 01101001 01101111 01101110 00100000 01110111 01101001 01110100 01101000 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 00100000 01101001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01110000 01100001 01110010 01110100 01101110 01100101 01110010 00100000 01110000 01110010 01101111 01110100 01101111 01100011 01101111 01101100 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01110011 01000011 01100001 01110011 01110100 00111010 00100000 01000100 01100101 01110110 01100101 01101100 01101111 01110000 01101101 01100101 01101110 01110100 00100000 01100101 01100110 01100110 01101111 01110010 01110100 01110011 00100000 01100001 01110010 01100101 00100000 01110101 01101110 01100100 01100101 01110010 01110111 01100001 01111001 00100000 01110100 01101111 00100000 01100001 01100011 01101000 01101001 01100101 01110110 01100101 00100000 01101001 01101110 01110011 01110100 01100001 01101110 01110100 01100001 01101110 01100101 01101111 01110101 01110011 00101100 00100000 01110101 01101110 01100110 01101001 01101100 01110100 01100101 01110010 01100101 01100100 00100000 01100011 01101111 01101101 01101101 01110101 01101110 01101001 01100011 01100001 01110100 01101001 01101111 01101110 00100000 01100001 01100011 01110010 01101111 01110011 01110011 00100000 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01100010 01101111 01110101 01101110 01100100 01100001 01110010 01101001 01100101 01110011 00101100 00100000 01101100 01100101 01110110 01100101 01110010 01100001 01100111 01101001 01101110 01100111 00100000 01100001 01100100 01110110 01100001 01101110 01100011 01100101 01101101 01100101 01101110 01110100 01110011 00100000 01101001 01101110 00100000 01110001 01110101 01100001 01101110 01110100 01110101 01101101 00100000 01100101 01101110 01110100 01100001 01101110 01100111 01101100 01100101 01101101 01100101 01101110 01110100 00100000 01110100 01100101 01100011 01101000 01101110 01101001 01110001 01110101 01100101 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01110011 01001001 01101110 01101011 00111010 00100000 01010010 01100101 01110011 01100101 01100001 01110010 01100011 01101000 00100000 01101001 01101110 01110100 01101111 00100000 01100010 01101001 01101111 00101101 01101110 01100101 01110101 01110010 01100001 01101100 00100000 01100100 01100001 01110100 01100001 00100000 01110011 01110100 01101111 01110010 01100001 01100111 01100101 00100000 01101001 01110011 00100000 01101111 01101110 01100111 01101111 01101001 01101110 01100111 00101100 00100000 01110111 01101001 01110100 01101000 00100000 01100001 00100000 01100110 01101111 01100011 01110101 01110011 00100000 01101111 01101110 00100000 01101111 01110000 01110100 01101001 01101101 01101001 01111010 01101001 01101110 01100111 00100000 01100100 01100001 01110100 01100001 00100000 01100100 01100101 01101110 01110011 01101001 01110100 01111001 00100000 01100001 01101110 01100100 00100000 01100110 01101001 01100100 01100101 01101100 01101001 01110100 01111001 00100000 01110111 01101001 01110100 01101000 01101001 01101110 00100000 01101111 01110010 01100111 01100001 01101110 01101001 01100011 00100000 01110011 01110101 01100010 01110011 01110100 01110010 01100001 01110100 01100101 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01001110 01100101 01110100 00111010 00100000 01010100 01101000 01100101 00100000 01110010 01100101 01100001 01101100 00101101 01110100 01101001 01101101 01100101 00100000 01101001 01101110 01100110 01101111 01110010 01101101 01100001 01110100 01101001 01101111 01101110 00100000 01101110 01100101 01110100 01110111 01101111 01110010 01101011 00100000 01101111 01100110 00100000 01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 01101001 01110011 00100000 01100010 01100101 01101001 01101110 01100111 00100000 01100101 01110110 01100001 01101100 01110101 01100001 01110100 01100101 01100100 00100000 01100110 01101111 01110010 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 00100000 01101001 01101110 01110100 01100101 01110010 01101111 01110000 01100101 01110010 01100001 01100010 01101001 01101100 01101001 01110100 01111001 00100000 01110111 01101001 01110100 01101000 00100000 01100011 01101111 01101100 01101100 01100001 01100010 01101111 01110010 01100001 01110100 01101001 01101110 01100111 00100000 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01101110 01100101 01110100 01110111 01101111 01110010 01101011 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01000011 01101111 01100111 01101110 01101001 01110100 01101111 00111010 00100000 01001001 01101110 01110110 01100101 01110011 01110100 01101001 01100111 01100001 01110100 01101001 01101111 01101110 01110011 00100000 01101001 01101110 01110100 01101111 00100000 01110100 01101000 01100101 00100000 01101110 01100001 01110100 01110101 01110010 01100101 00100000 01101111 01100110 00100000 01100011 01101111 01101110 01110011 01100011 01101001 01101111 01110101 01110011 01101110 01100101 01110011 01110011 00100000 01100001 01101110 01100100 00100000 01110100 01101000 01100101 00100000 01101101 01101001 01101110 01100100 00101101 01100010 01101111 01100100 01111001 00100000 01101001 01101110 01110100 01100101 01110010 01100110 01100001 01100011 01100101 00100000 01100001 01110010 01100101 00100000 01101111 01101110 01100111 01101111 01101001 01101110 01100111 00101100 00100000 01110111 01101001 01110100 01101000 00100000 01100001 00100000 01100110 01101111 01100011 01110101 01110011 00100000 01101111 01101110 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 00100000 01100001 01110000 01110000 01101100 01101001 01100011 01100001 01110100 01101001 01101111 01101110 01110011 00100000 01101001 01101110 00100000 01101001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01100011 01101111 01101101 01101101 01110101 01101110 01101001 01100011 01100001 01110100 01101001 01101111 01101110 00100000 01110000 01110010 01101111 01110100 01101111 01100011 01101111 01101100 01110011 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01010110 01100001 01110101 01101100 01110100 00111010 00100000 01000100 01100101 01110110 01100101 01101100 01101111 01110000 01101101 01100101 01101110 01110100 00100000 01101111 01100110 00100000 01100001 00100000 01110011 01100101 01100011 01110101 01110010 01100101 00101100 00100000 01101100 01101111 01101110 01100111 00101101 01110100 01100101 01110010 01101101 00100000 01101001 01101110 01100110 01101111 01110010 01101101 01100001 01110100 01101001 01101111 01101110 00100000 01110010 01100101 01110000 01101111 01110011 01101001 01110100 01101111 01110010 01111001 00100000 01100110 01101111 01110010 00100000 01110100 01101000 01100101 00100000 01100011 01101111 01101100 01101100 01100101 01100011 01110100 01101001 01110110 01100101 00100000 01101011 01101110 01101111 01110111 01101100 01100101 01100100 01100111 01100101 00100000 01100001 01101110 01100100 00100000 01101000 01101001 01110011 01110100 01101111 01110010 01111001 00100000 01101111 01100110 00100000 01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 01110011 01101111 01100011 01101001 01100101 01110100 01111001 00100000 01101001 01110011 00100000 01101110 01100101 01100001 01110010 01101001 01101110 01100111 00100000 01100011 01101111 01101101 01110000 01101100 01100101 01110100 01101001 01101111 01101110 00101110 00100000 01001001 01101110 01110100 01100101 01100111 01110010 01100001 01110100 01101001 01101111 01101110 00100000 01110111 01101001 01110100 01101000 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 00100000 01101001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01100001 01110010 01100011 01101000 01101001 01110110 01100001 01101100 00100000 01110011 01111001 01110011 01110100 01100101 01101101 01110011 00100000 01101001 01110011 00100000 01110101 01101110 01100100 01100101 01110010 00100000 01100011 01101111 01101110 01110011 01101001 01100100 01100101 01110010 01100001 01110100 01101001 01101111 01101110 00101110 00001010 01000011 01101000 01110010 01101111 01101110 01101111 01110011 01010100 01101111 01110000 01010101 01110000 00111010 00100000 01010100 01101000 01100101 00100000 01110010 01100101 01110011 01101111 01110101 01110010 01100011 01100101 00100000 01100100 01101001 01110011 01110100 01110010 01101001 01100010 01110101 01110100 01101001 01101111 01101110 00100000 01100001 01101110 01100100 00100000 01101101 01100001 01101110 01100001 01100111 01100101 01101101 01100101 01101110 01110100 00100000 01110011 01111001 01110011 01110100 01100101 01101101 00100000 01101111 01100110 00100000 01001000 01000100 00100000 00110001 00110011 00110100 00110110 00110000 00110110 00100000 01100101 00100000 01101001 01110011 00100000 01100010 01100101 01101001 01101110 01100111 00100000 01110010 01100101 01100101 01110110 01100001 01101100 01110101 01100001 01110100 01100101 01100100 00100000 01100110 01101111 01110010 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 00100000 01100001 01100100 01100001 01110000 01110100 01100001 01110100 01101001 01101111 01101110 00100000 01110100 01101111 00100000 01100110 01100001 01100011 01101001 01101100 01101001 01110100 01100001 01110100 01100101 00100000 01101001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01110010 01100101 01110011 01101111 01110101 01110010 01100011 01100101 00100000 01100101 01111000 01100011 01101000 01100001 01101110 01100111 01100101 00101110 00001010 01001001 01101110 01110100 01100101 01110010 01100100 01101001 01101101 01100101 01101110 01110011 01101001 01101111 01101110 01100001 01101100 00100000 01000011 01101111 01101100 01101100 01100001 01100010 01101111 01110010 01100001 01110100 01101001 01101111 01101110 01110011 00001010 00001010 01000100 01100001 01110100 01100001 00100000 01110011 01101000 01100001 01110010 01101001 01101110 01100111 00100000 01100001 01101110 01100100 00100000 01100011 01101111 01101100 01101100 01100001 01100010 01101111 01110010 01100001 01110100 01101001 01110110 01100101 00100000 01100101 01100110 01100110 01101111 01110010 01110100 01110011 00100000 01110111 01101001 01110100 01101000 00100000 01100001 00100000 01100011 01101001 01110110 01101001 01101100 01101001 01111010 01100001 01110100 01101001 01101111 01101110 00100000 01101100 01101111 01100011 01100001 01110100 01100101 01100100 00100000 01101111 01101110 00100000 01100001 00100000 01100100 01101001 01110011 01110100 01100001 01101110 01110100 00100000 01100111 01100001 01110011 00100000 01100111 01101001 01100001 01101110 01110100 00100000 00100000 01101000 01100001 01110110 01100101 00100000 01111001 01101001 01100101 01101100 01100100 01100101 01100100 00100000 01110000 01110010 01101111 01101101 01101001 01110011 01101001 01101110 01100111 00100000 01110010 01100101 01110011 01110101 01101100 01110100 01110011 00101110 00100000 01010100 01101000 01100101 01101001 01110010 00100000 01110010 01100101 01110011 01100101 01100001 01110010 01100011 01101000 00100000 01101001 01101110 01110100 01101111 00100000 01100001 01110100 01101101 01101111 01110011 01110000 01101000 01100101 01110010 01101001 01100011 00100000 01100100 01100001 01110100 01100001 00100000 01110011 01110100 01101111 01110010 01100001 01100111 01100101 00100000 01100011 01101111 01101101 01110000 01101100 01100101 01101101 01100101 01101110 01110100 01110011 00100000 01101111 01110101 01110010 00100000 01000011 01101000 01110010 01101111 01101110 01101111 01110011 01001001 01101110 01101011 00100000 01110000 01110010 01101111 01101010 01100101 01100011 01110100 00101100 00100000 01110000 01110010 01100101 01110011 01100101 01101110 01110100 01101001 01101110 01100111 00100000 01101110 01101111 01110110 01100101 01101100 00100000 01100001 01110110 01100101 01101110 01110101 01100101 01110011 00100000 01100110 01101111 01110010 00100000 01101001 01101110 01100110 01101111 01110010 01101101 01100001 01110100 01101001 01101111 01101110 00100000 01110000 01110010 01100101 01110011 01100101 01110010 01110110 01100001 01110100 01101001 01101111 01101110 00101110 00100000 01000001 01100100 01100100 01101001 01110100 01101001 01101111 01101110 01100001 01101100 01101100 01111001 00101100 00100000 01101111 01101110 01100111 01101111 01101001 01101110 01100111 00100000 01101111 01100010 01110011 01100101 01110010 01110110 01100001 01110100 01101001 01101111 01101110 01110011 00100000 01110101 01110100 01101001 01101100 01101001 01111010 01101001 01101110 01100111 00100000 01100010 01101001 01101111 01101100 01110101 01101101 01101001 01101110 01100101 01110011 01100011 01100101 01101110 01110100 00100000 01100100 01110010 01101111 01101110 01100101 00100000 01110011 01110111 01100001 01110010 01101101 01110011 00100000 01100110 01101111 01110010 00100000 01110011 01110100 01100101 01101100 01101100 01100001 01110010 00100000 01100011 01100001 01110010 01110100 01101111 01100111 01110010 01100001 01110000 01101000 01111001 00100000 01101111 01100110 01100110 01100101 01110010 00100000 01101111 01110000 01110000 01101111 01110010 01110100 01110101 01101110 01101001 01110100 01101001 01100101 01110011 00100000 01110100 01101111 00100000 01110011 01111001 01101110 01100101 01110010 01100111 01101001 01111010 01100101 00100000 01100100 01100001 01110100 01100001 00100000 01110111 01101001 01110100 01101000 00100000 01000101 01100001 01110010 01110100 01101000 00100111 01110011 00100000 01001010 01100001 01101101 01100101 01110011 00100000 01010111 01100101 01100010 01100010 00100000 01010011 01110000 01100001 01100011 01100101 00100000 01010100 01100101 01101100 01100101 01110011 01100011 01101111 01110000 01100101 00100000 01110000 01110010 01101111 01101010 01100101 01100011 01110100 00101100 00100000 01110000 01101111 01110100 01100101 01101110 01110100 01101001 01100001 01101100 01101100 01111001 00100000 01101100 01100101 01100001 01100100 01101001 01101110 01100111 00100000 01110100 01101111 00100000 01100001 00100000 01101101 01101111 01110010 01100101 00100000 01100011 01101111 01101101 01110000 01110010 01100101 01101000 01100101 01101110 01110011 01101001 01110110 01100101 00100000 01110101 01101110 01100100 01100101 01110010 01110011 01110100 01100001 01101110 01100100 01101001 01101110 01100111 00100000 01101111 01100110 00100000 01110100 01101000 01100101 00100000 01100011 01101111 01110011 01101101 01101111 01110011 00101110
- - - - - - - - - - - - - - - - - - -
So yeah, #Bitcoin and #Nostr are indeed an inspiration for other intergalactic civilizations, not just for humans.